springboot极大的简化了SSM的搭建和开发,使我们能够专注于业务层开发而无需被繁琐的配置所困扰。
1. 创建springboot项目
创建项目时选择spring initializr,下一步下一步,到了dependency时,选择自已需要的依赖。现在我们要做web工程,就选择web→spring web
pom文件可能会遇到两个问题:
spring-boot-starter-parent飘红
File→invalidate cache/restart
spring-boot-maven-plugin飘红
指定其版本号,跟org.springframework.boot的一样即可
1 2 3 4 5 6 7 8 9
| <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>2.4.3</version> </plugin> </plugins> </build>
|
【之后我们所有的代码都必须放在SpringBoot启动类所在目录中】
2. 核心配置文件application.properties
1 2 3 4 5 6 7 8
| server.port=8080
server.servlet.context-path=/demo
mybatis.mapper-location=classpath:mapper/*.xml
|
核心配置文件只能有一个,也可能是yml结尾的,它们区别仅仅是书写格式不同。
如果复制别人的配置文件,一定要注意格式,把空格什么的删干净(尤其是行尾空格)。
3. 多环境下核心配置文件的使用
工作中可能有多个环境: 开发环境、测试环境、准生产环境和生产环境。
比如开发环境下我们用localhost就可查看网页,而到了测试环境一般就要用真实的ip地址来查看网页,这时配置文件就不同了。生产环境和测试环境的配置又不一样了。springboot提供了配置文件的切换功能,这样就不用换一个环境就改一次配置文件。
- 创建多个properties,名称中要体现当前是什么环境(格式如图,必须遵守)
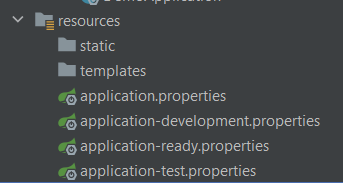
针对不同环境写好不同的配置文件
- 主核心配置文件中指定当前应用的配置文件
1 2
| #使用哪个配置文件 spring.profiles.active=test
|
yml格式配置类似。
4. 获取自定义配置
【spring核心配置文件中尽量不要有中文】
4.1 通过value注解逐个获取
配置文件中的数据是以键值对的形式存在的,我们可以自定义属性
然后在代码中使用注解的形式来获取该属性
1 2
| @Value("${age}") private int age;
|
4.2 通过对象获取
首先在配置文件中定义(必须有前缀)
1 2
| student.name=tom student.age=18
|
然后将实体类注册
1 2 3
| @Component @ConfigurationProperties(prefix = "student") public class Student {
|
最后直接用autowired注解即可获取对象
1 2
| @Autowired private Student student;
|
5. 集成jsp
- 创建webapp文件夹
首先在main目录下创建一个与java同级的webapp文件夹,然后打开project structure
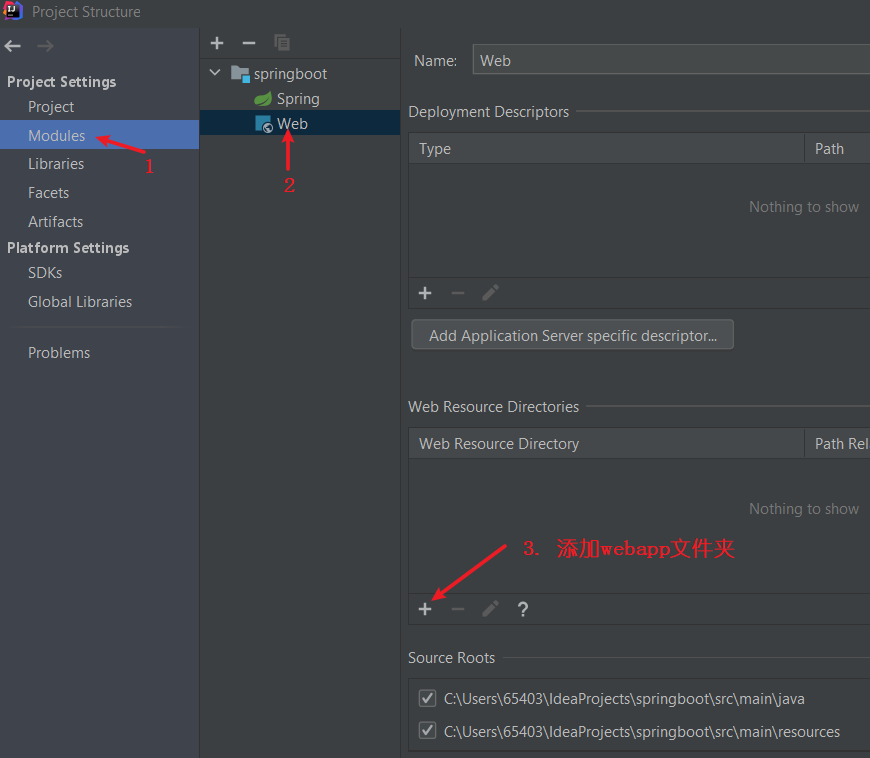
然后点击create artifact,ok
- pom文件配置
springboot默认前端引擎尾thymeleaf,要将其更改为jsp。在build标签中添加
1 2 3 4 5 6 7 8 9 10 11 12 13
| <resources> <resource> <directory>src/main/webapp</directory> <targetPath>META-INF/resources</targetPath> <includes> <include>*.*</include> </includes> </resource> </resources>
|
然后刷新项目。
- 在springboot中配置视图解析器
1 2
| spring.mvc.view.prefix=/ spring.mvc.view.suffix=.jsp
|
接下来就跟之前一样了,用@RequestMapping给资源绑定方法,在webapp目录下创建jsp页面。
6. 集成MyBatis
- 添加mybatis依赖,添加mysql驱动
mybatis有两个依赖,一个mybatis,一个spring集成mybatis。springboot将它们整合成了一个起步依赖。
1 2 3 4 5 6 7 8 9 10
| <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
<dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.4</version> </dependency>
|
做这一步的时候我们会发现有些依赖直接就加了,不需要下载,这是因为springboot中已经集成了。
6.1 MyBatis逆向工程
通过扫描数据库倒推出要创建的MyBatis文件,就不用我们自己创建mapper.xml,实体类等。
- 在项目的根目录下创建GeneratorMapper.xml文件,并复制下列内容
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70
| <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration>
<classPathEntry location="C:\Users\65403\.m2\repository\mysql\mysql-connector-java\8.0.23\mysql-connector-java-8.0.23.jar" />
<context id="msqlTables" targetRuntime="MyBatis3">
<plugin type="org.mybatis.generator.plugins.SerializablePlugin"></plugin>
<commentGenerator> <property name="suppressAllComments" value="true"/> </commentGenerator>
<jdbcConnection connectionURL="jdbc:mysql://localhost:3306/test?serverTimezone=Asia/Shanghai" driverClass="com.mysql.cj.jdbc.Driver" userId="root" password=""> <property name="nullCatalogMeansCurrent" value="true"/> </jdbcConnection>
<javaTypeResolver> <property name="forceBigDecimals" value="false" /> </javaTypeResolver>
<javaModelGenerator targetPackage="com.aaron.demo.domain" targetProject="src/main/java"> <property name="enableSubPackages" value="true"/> <property name="trimStrings" value="true" /> </javaModelGenerator>
<sqlMapGenerator targetPackage="mapper" targetProject="src/main/resources"> <property name="enableSubPackages" value="true"/> </sqlMapGenerator>
<javaClientGenerator type="XMLMAPPER" targetPackage="com.aaron.demo.mapper" targetProject="src/main/java"> <property name="enableSubPackages" value="true"/> </javaClientGenerator>
<table tableName="student" domainObjectName="Student" enableCountByExample="false" enableUpdateByExample="false" enableDeleteByExample="false" enableSelectByExample="false" selectByExampleQueryId="false" > <property name="useActualColumnNames" value="false"/> </table> </context> </generatorConfiguration>
|
- 在pom中plugins标签下添加以下插件
1 2 3 4 5 6 7 8 9 10 11 12
| <plugin> <groupId>org.mybatis.generator</groupId> <artifactId>mybatis-generator-maven-plugin</artifactId> <version>1.3.6</version> <configuration> <configurationFile>GeneratorMapper.xml</configurationFile> <verbose>true</verbose> <overwrite>true</overwrite> </configuration> </plugin>
|
点击右侧maven标签,刷新,然后找到plugin→mybatis.generator→双击generate
在springboot入口函数上面加上注解MapperScan,这样后面就可以autowire
1 2 3 4 5 6 7 8
| @SpringBootApplication @MapperScan(basePackages = "com.aaron.demo.mapper") public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
|
在核心配置文件中添加数据库信息
1 2 3 4
| spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/test?serverTimezone=Asia/Shanghai spring.datasource.username=root spring.datasource.password=
|
如果mapper文件不是放在resources目录下而是在java目录下,这时直接运行会报binding exception,原因是mapper文件放到java目录下,而框架默认不扫描java目录下的xml配置文件。去pom中将java目录指定为resources文件夹即可(build标签中的resources标签中添加)
1 2 3 4 5 6
| <resource> <directory>src/main/java</directory> <includes> <include>**/*.xml</include> </includes> </resource>
|
然后reload project后就可以正常运行了。
6.2 逆向生成接口方法详解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public interface StudentMapper {
int deleteByPrimaryKey(Integer id);
int insert(Student record);
int insertSelective(Student record);
Student selectByPrimaryKey(Integer id);
int updateByPrimaryKeySelective(Student record);
int updateByPrimaryKey(Student record); }
|
6.3 事务
在服务层要使用事务的方法上注解@Transactional
即可,然后该方法要么全部执行,要么都不执行(如果中途出现错误)。
7. 拦截器
定义拦截器类,实现HandlerInterceptor接口
创建interceptor包,在里面创建需要的拦截器类。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class StudentInterceptor implements HandlerInterceptor { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { return false; }
@Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception {
}
@Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
} }
|
创建一个配置类(以前是在配置文件中配置,现在创建类)
创建config包用来存放配置类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| @Configuration public class InterceptorConfig implements WebMvcConfigurer { @Override public void addInterceptors(InterceptorRegistry registry) { String[] addPathPatterns = { "/student/**" }; String[] excludePathPatterns = { "/student/page" }; registry.addInterceptor(new StudentInterceptor()) .addPathPatterns(addPathPatterns) .excludePathPatterns(excludePathPatterns); } }
|
8. Servlet
直接在springboot主方法上加注解
1 2 3 4 5 6 7 8 9
| @ServletComponentScan public class DemoApplication {
public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); }
}
|
然后servlet上加注解
@WebServlet(urlPatterns = "/path")
9. Filter
- 声明过滤器类,实现Filter接口(servlet包下),加上WebFilter注解
1 2 3 4 5 6 7
| @WebFilter(urlPatterns = "myFilter") public class MyFilter implements Filter { @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
} }
|
- 在springboot主方法加上注解,自动扫描目标目录下所有Filter
1 2 3 4 5 6 7 8 9
| @ServletComponentScan public class DemoApplication {
public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); }
}
|
10. 打包与部署
10.1 打war包
在pom文件中加入
<packaging> war </packaging>
如果有jsp文件,要在pom文件中指定
1 2 3 4 5 6 7 8 9 10 11 12 13
| <resources> <resource> <directory>src/main/webapp</directory> <targetPath>META-INF/resources</targetPath> <includes> <include>*.*</include> </includes> </resource> </resources>
|
在pom文件中,build标签下,指定生成war包的名称
<finalName> myWar </finalName>
更改springboot主方法
1 2 3 4 5 6 7 8 9 10 11 12 13
| @SpringBootApplication public class DemoApplication extends SpringBootServletInitializer {
public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); }
@Override protected SpringApplicationBuilder configure(SpringApplicationBuilder builder) { return builder.sources(DemoApplication.class); } }
|
点击右侧maven,选中当前项目→lifecycle→package
左侧,target目录下找到:项目名.war文件,复制,然后关闭当前项目
下载tomcat,将war包复制到其webapps目录下
进入bin目录,点击startup.bat启动tomcat
注意,打了war包后项目中原核心配置文件中的上下文根和端口号失效,之后以本地tomcat为准。
10.2 打jar包
同war包,除了指定<packaging> war </packaging>
这一步。
另外还要指定org.springframework.boot的maven-plugin版本号为1.4.2.RELEASE
运行jar包不需要放到tomcat文件夹中,只需cmd执行java -jar {jar包}
即可。
且jar包的上下文根和端口号与项目中核心配置文件一致。